Mastering Asynchronous JavaScript with jQuery Wait
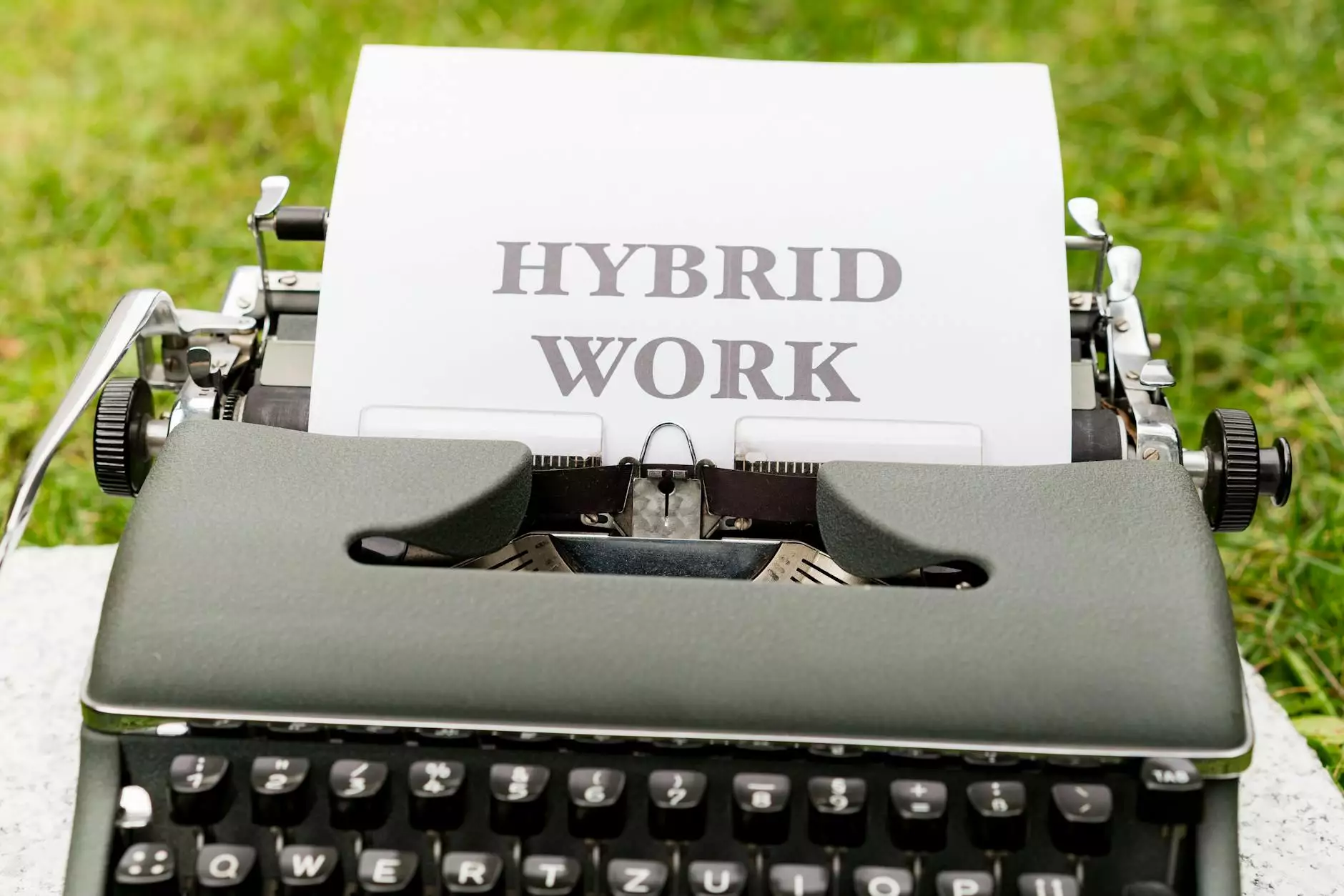
In today’s digital landscape, businesses are increasingly leveraging web technologies to improve their services and customer satisfaction. One crucial aspect of web development is managing asynchronous events, and one powerful tool to achieve this is the jQuery library. jQuery simplifies the process of handling JavaScript functionalities, particularly with its jQuery wait feature, which allows developers to create seamless, interactive experiences. In this article, we'll deep dive into the jQuery wait functionality and how it can transform your web development projects, ultimately boosting your business's online presence and customer engagement.
Understanding Asynchronous JavaScript
Before diving into the specifics of jQuery wait, it's vital to grasp the concept of asynchronous JavaScript. Asynchronous programming allows JavaScript to execute non-blocking operations, meaning it can handle multiple tasks at once without freezing the user interface. This is particularly important when fetching data from APIs, loading images, or waiting for certain user actions.
- Non-blocking operations: These allow the browser to remain responsive while performing tasks in the background.
- Event-driven architecture: JavaScript can respond to various events without interrupting the flow of the application.
- Callbacks and promises: These structures help manage asynchronous code, making it easier to work with multiple operations.
The Role of jQuery in Web Development
jQuery is a fast, small, and feature-rich JavaScript library that simplifies things like HTML document traversal and manipulation, event handling, and animation. It provides an easy-to-use API that works across a multitude of browsers, enabling developers to create robust applications quickly. jQuery's chaining methods and built-in AJAX functionalities make it exceptionally powerful for web development.
Why Use jQuery?
- Simplicity: jQuery’s syntax is simple and easy to understand, allowing developers to write less code and achieve more.
- Cross-browser compatibility: It handles differences between browsers seamlessly, enabling a smoother development process.
- Extensive plugins: jQuery has a rich ecosystem of plugins that extend its functionality, offering solutions for various needs.
Introducing jQuery Wait
The jQuery wait functionality is often used in scenarios where developers need to delay certain actions or wait for events to complete before executing subsequent code. This is particularly useful in creating animations, waiting for AJAX requests to finish, or implementing timed delays in user interactions.
Common Uses of jQuery Wait
- Animations: Control the timing of animations to create a smoother visual flow.
- AJAX Requests: Wait for responses from the server before proceeding with the application logic.
- Delayed Actions: Implement set timeouts for certain actions, such as displaying messages after a user event.
Implementing jQuery Wait in Your Projects
To harness the power of jQuery wait, you first need to ensure that jQuery is included in your project. You can do this by adding a script tag in your HTML code:
Basic Example of jQuery Wait
Here’s a simple example demonstrating the jQuery wait functionality:
$(document).ready(function() { $("#myButton").click(function() { $("#myDiv").fadeOut(1000, function() { // This code will run after the fading out is complete console.log("Div has faded out!"); $("#myDiv").fadeIn(1000); }); }); });In this example, when the button is clicked, the div will fade out, wait for one second (1000 milliseconds), and then fade back in. This showcases how jQuery wait works in a practical application.
Advanced Usage with AJAX
Asynchronous calls to APIs are a fundamental part of web applications. jQuery’s AJAX capabilities paired with the jQuery wait functionality enable developers to create applications that fetch data without refreshing the page:
$.ajax({ url: 'https://api.example.com/data', type: 'GET', beforeSend: function() { $("#loader").show(); // Show loader before the request }, success: function(data) { $("#loader").hide(); // Hide loader once the data is received // Process the received data here console.log(data); }, error: function(error) { $("#loader").hide(); console.error("Error fetching data:", error); } });In this AJAX example, the loader appears before the request is sent and disappears once the data is received, ensuring the user is informed about the process taking place.
Creating Engaging User Experiences with jQuery Wait
In the age of user-centric design, providing engaging and fluid interactions is crucial for retaining visitors. The jQuery wait functionality plays a significant part in creating these interactions. Here are several strategies to enhance user experiences using jQuery:
Utilizing Timed Notifications
Notifications are essential in web applications for keeping users informed. Using jQuery wait, we can display notification messages for a limited time to enhance user engagement:
function showNotification(message) { $("#notification").text(message).fadeIn().delay(2000).fadeOut(); // Display notification for 2 seconds }This function will show a notification message, making it visible to the user for two seconds before fading it out.
Loading Spinners for Data Fetching
When data is being loaded via AJAX, incorporating a loading spinner can significantly enhance user feedback:
function loadData() { $("#loadingSpinner").show(); $.ajax({ url: 'https://api.example.com/data', success: function(data) { $("#loadingSpinner").hide(); // Process the received data } }); }This snippet displays a loading spinner while the data is being fetched, providing a clear visual cue that the application is busy and enhancing the overall user experience.
Best Practices for Using jQuery Wait
As with any powerful tool, there are best practices to consider when implementing jQuery wait functionalities in your projects:
- Optimize Performance: Ensure that animations and waits are not overly lengthy, which could lead to a frustrating user experience.
- Provide Feedback: Use visual cues like spinners or loading bars to inform users about ongoing processes.
- Be Mindful of User Actions: Prevent excessive waits during important user actions, which could lead to abandonment.
Conclusion
The functionality provided by jQuery wait is invaluable for modern web development, allowing for smoother interactions and enhanced user experiences. By mastering jQuery, businesses can improve their online offerings, ultimately fostering increased customer satisfaction and engagement. Whether it's through AJAX data fetching, timed notifications, or seamless animations, jQuery provides the tools necessary to build dynamic and responsive applications.
Prodjex.com specializes in IT services and computer repair, web design, and software development, empowering businesses to leverage the full potential of web technologies. Embrace the power of jQuery and unlock new possibilities for your digital presence!